There are several ways to call or run another application form within python code. In this article let us see a few of the commonly used methods. All these methods have their own advantages and disadvantages. Let us see them with examples.
Using subprocess.run method
The most commonly used way to call or run another application from Python code is by using the subprocess.run() method. This run() function is part of the subprocess module. subprocess.run() is available in Python version 3.5 and later. This method allows you to spawn new processes, connect to their input/output/error pipes, and obtain their return codes. subprocess.run() is the recommended approach for invoking the subprocess module. Here is a simple example to call an external application using subprocess.run().
import subprocess
# Run commands on terminal
subprocess.run(["ls", "-l"])
# Launch notepad with a text file
subprocess.run(["notepad","filename.txt"])
Here is another example to run an external command and capture the output and the return code. In subprocess.run(), by adding check=True, you can raise a subprocess.CalledProcessError if the command returns a non-zero exit code, indicating an error. by adding capture_output=True, you can capture the output to a variable.
import subprocess
# Run commands on terminal and get the result back
result = subprocess.run(["ls", "-l"], check=True, capture_output=True)
print(f"\nReturn code: {result.returncode}")
print(f"\nResult: {result}")
Result:
Return code: 0
Result: CompletedProcess(args=['ls', '-l'], returncode=0,
stdout=b'total 796\n-rwxr-xr-x 1 beaulin beaulin 644
Jul 11 2019 class.py\ndrwxr-xr-x 6 beaulin beaulin
4096 Dec 14 21:14 env\n-rw-rw-r-- 1 beaulin beaulin
0 Feb 15 10:22 filename.txt\n', stderr='')
Using subprocess.call method
If you are using Python version older than 3.5, then you can use the subprocess.call() method. In Python 3.5 and later, subprocess.call() is deprecated in favor of the more feature-rich and flexible subprocess.run() function. subprocess.run() is recommended for more flexibility.
import subprocess
# Run commands on terminal
subprocess.call(["ls", "-l"])
# Launch notepad with a text file
subprocess.call(["notepad","filename.txt"])
Using subprocess.Popen method
subprocess.Popen() is similar to subprocess.run(). However it is a low-level function that provides more control over the subprocess. This allows you to interact with its input and output streams in a non-blocking way. So, this method is suitable to be used in more complex scenarios. Here is an example:
import subprocess
popObject = subprocess.Popen(['ls', '-l'], stdout=subprocess.PIPE, text=True)
result, _ = popObject.communicate()
return_code = popObject.returncode
print(f"\nReturn code: {return_code}")
print(f"\nOutput: {result}")
Result:
Return code: 0
Output: total 796
-rwxr-xr-x 1 beaulin beaulin 644 Jul 11 2019 class.py
drwxr-xr-x 6 beaulin beaulin 4096 Dec 14 21:14 env
-rw-rw-r-- 1 beaulin beaulin 0 Feb 15 10:22 filename.txt
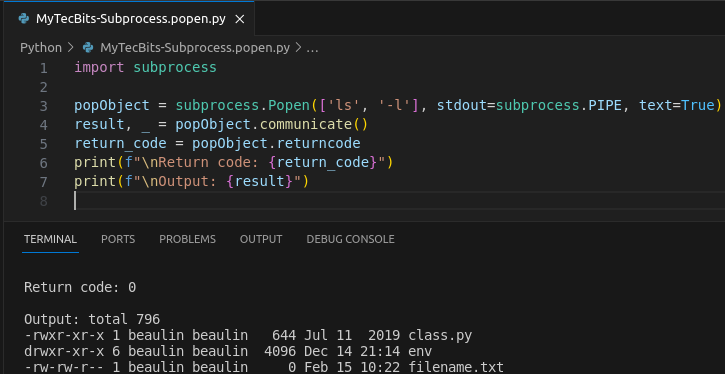
Other methods
Other methods like os.system, os.popen, ect. can also be used to run another application from python code. However, these are not recommended as they are outdated and the subprocess module provides more powerful and flexible features for calling another application and retrieving their results.
Reference
- More about subprocess.run() at Python Docs.
- More about subprocess.call() at Python Docs.
- More about subprocess.Popen() at Python Docs.