In Python, you can iterate through a dictionary using loops such as for loops. In the for loop itself, you can iterate through keys, values, or key-value pairs (items) of the dictionary. Let us see these methods one by one.
1. Using KEYS in FOR loop
By default while iterating through a dictionary using a for loop, the loop will iterate through the keys. So, you don’t need to specify the dictionary-specific method keys(). Here is an example:
my_dictionary = {'a': 1001, 'b': 1002, 'c': 1003, 'd': 1004}
# Iterating through keys
for key in my_dictionary:
print(key)
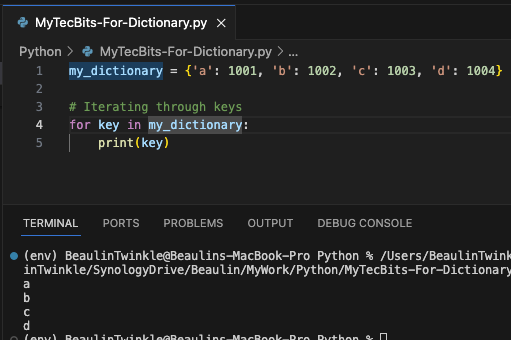
2. Using VALUES in FOR loop
If you want to iterate through the values of the dictionary, then use the dictionary-specific method values(). Here is an example:
my_dictionary = {'a': 1001, 'b': 1002, 'c': 1003, 'd': 1004}
# Iterating through values
for value in my_dictionary.values():
print(value)
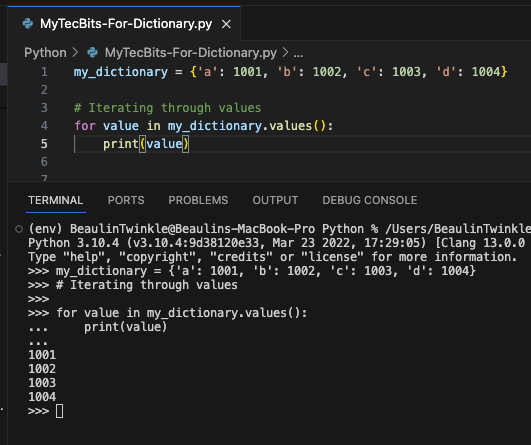
3. Using KEY-VALUE pairs in FOR loop
In case, if you want to fetch both the key and the value while iterating through a dictionary, then use the method like items() in python version 3.x and iteritems() in version 2.x. Here are the examples:
For Python version 3.x
my_dictionary = {'a': 1001, 'b': 1002, 'c': 1003, 'd': 1004}
# Iterating through key-value pairs
for key, value in my_dictionary.items():
print(f"Key: {key}, Value: {value}")
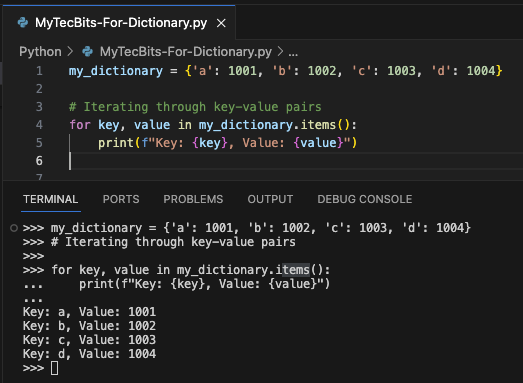
For Python version 2.x
my_dictionary = {'a': 1001, 'b': 1002, 'c': 1003, 'd': 1004}
# Iterating through key-value pairs
for key, value in my_dictionary.iteritems():
print("Key: ", key ," Value: ", value)
Related Articles
- How to access the index while iterating a sequence with a for loop in python?
- How to find the index of an item in a list in python?
Reference
- Read more about Python’s looping techniques at Python Docs.