Lately I came across a requirement to check if the string value is a float and if float then parse it to a float value. To check and parse a string to float, I wrote two simple functions. One of the function named isFloat, check if the string value is float or not, if float it will return true, else false. The other function, named convertToFloat, uses the isFloat function to check the string and if it is a float then it parses the string to a float and returns it. Here is the sample code.
def isFloat(str_val):
try:
float(str_val)
return True
except ValueError:
return False
def convertToFloat(str_val):
if isFloat(str_val):
fl_result = float(str_val)
return fl_result
else:
return str_val + " is not a float."
print(convertToFloat("1234.56789"))
print(convertToFloat("25234"))
print(convertToFloat("ABCD"))
print(convertToFloat("00"))
print(convertToFloat(".00"))
print(convertToFloat("23e2"))
print(convertToFloat("-54"))
print(convertToFloat("1234..56789"))
Result
1234.56789
25234.0
ABCD is not a float.
0.0
0.0
2300.0
-54.0
1234..56789 is not a float.
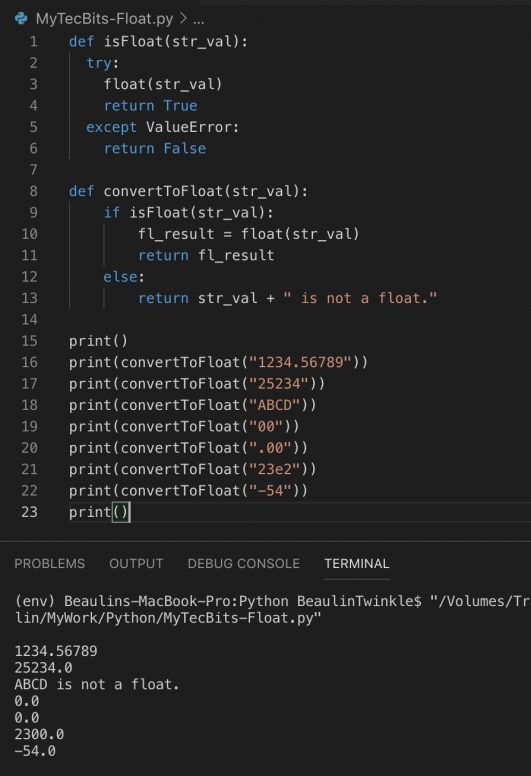
Read my other article on extracting numbers from a string in Python programming.
Reference
- Question about checking if a string value can be parsed to float in stackoverflow.