Generating random number in C# is required in several situations like simulations, testing, etc. In C#.NET, there is a built-in class called Random under the System namespace. Using this Random class we can generate random integers, floating-point numbers, bytes, etc. Let’s see them in detail with an example.
Sample Program
using System;
class Program
{
static void Main()
{
// Create an instance of Random
Random randObj = new Random();
// Generating random integers
int randInt1 = randObj.Next();
int randInt2 = randObj.Next(50);
int randInt3 = randObj.Next(50, 101);
// Generating random bytes
byte[] byteArray = new byte[5];
randObj.NextBytes(byteArray);
// Generating random floating points
double randDbl1 = randObj.NextDouble();
double randDbl2 = randObj.NextDouble() * 5;
// Output
Console.WriteLine("Random integer value: Ranging from 0 to Int32.MaxValue: " + randInt1);
Console.WriteLine("Random integer value: Ranging from 0 to 49: " + randInt2);
Console.WriteLine("Random integer value: Ranging from 51 to 100: " + randInt3 + "\n");
Console.Write("Random byte values: ");
foreach (byte b in byteArray)
{
Console.Write(b + " ");
}
Console.WriteLine("\n\nRandom floating point values: Ranging from 0.0 to 1.0: " + randDbl1);
Console.WriteLine("Random floating point values: Ranging from 0.0 to 5.0: " + randDbl2);
}
}
Output
Random integer value: Ranging from 0 to Int32.MaxValue: 1257468394
Random integer value: Ranging from 0 to 49: 20
Random integer value: Ranging from 51 to 100: 69
Random byte values: 65 96 234 118 23
Random floating point values: Ranging from 0.0 to 1.0: 0.03225536997811018
Random floating point values: Ranging from 0.0 to 5.0: 3.4793799070858915
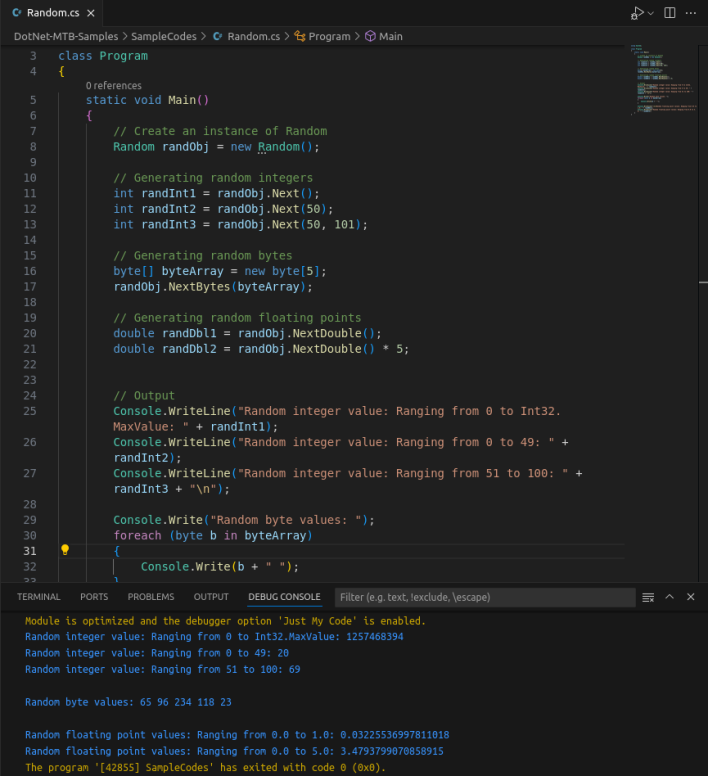
Creating an instance
In the above example the first thing I’ve done is create an instance of the Random class.
Random randObj = new Random();
Random integer values
Then using the random instance, I’ve generated a random number using the Next() method. By default the Next() method generates random number ranging from 0 to the maximum of integer, i.e. Int32.MaxValue. However, if you want to reduce the maximum to your required value, then assign a max value to Next() like Next(50), which will generate a random integer between 0 to 49. Instead of the range starting from 0, if you want a specific range then specify the range in Next() like Next(50,101), which will generate a random integer between 51 to 100.
Random floating-point values
To generate random floating-point values, I’ve used the NextDouble() method. This method generates a random floating point between 0 and 1. Instead of limiting the max value to 1, if you want to get a random floating-point more than 1, then multiply the result of the NextDouble() method with the maximum value. In my example I’ve multiplied with 5 to generate a random floating point between 0 and 5
double randDbl2 = randObj.NextDouble() * 5;
Random byte values
To generate random byte values, I’ve used the NextBytes() method with an instance of a byte array.
byte[] byteArray = new byte[5];
randObj.NextBytes(byteArray);
Reference
- More about the Random() class at Microsoft Docs.
Related Articles
- Generating random numbers in Python language.
- Generating random numbers in SQL Server.