In python, you can index a string like a list. The first character is considered as index 0. Using this indexing technique we can slice to get a sub-string from a string. Here are few of the slicing techniques we can use to get sub-string.
string[: to_char] --> From starting to few characters
string[-number_of_char :] --> From last few characters
string[after_char :] --> From a specific character to the end
string[after_char : to_char] --> From a specific character to another in-between the string
Let’s see how to get the sub string with examples.
From starting to few characters
If you want the sub-string form the beginning of a string till certain character, use the slicing technique string[: to_char]. For example, to get the first two characters of a string as a sub string then use string[:2]. Here is a example.
# Initiate a string
a_string = "My Tec Bits"
# slice
sub_string = a_string[:2]
print(sub_string)
Output
My
From last few characters
To get the last few characters of a string then use the slicing technique string[-number_of_char :]. For example, if you want to get the last 4 characters of a string as a substring then use string[-4:]. Here is a example.
# Initiate a string
a_string = "My Tec Bits"
# slice
sub_string = a_string[-4:]
print(sub_string)
Output
Bits
From a specific character to the end
To get the last few characters of a string after a specific character then use the slicing technique string[after_char :]. For example, if you want to get a sub-string starting after the 4th char of the string to the end then use string[4:]. This will give a substring from the fifth character to the end. Here is a example.
# Initiate a string
a_string = "My Tec Bits"
# slice
sub_string = a_string[4:]
print(sub_string)
Output
ec Bits
From a specific character to another in-between the string
To get a sub-string from a string after a specific character to another specific character use the slicing technique string[after_char : to_char]. For example, if you want to get a sub-string starting after the 4th char of the string to the 9th char then use string[4:9]. Here is a example.
# Initiate a string
a_string = "My Tec Bits"
# slice
sub_string = a_string[4:9]
print(sub_string)
Output
ec Bi
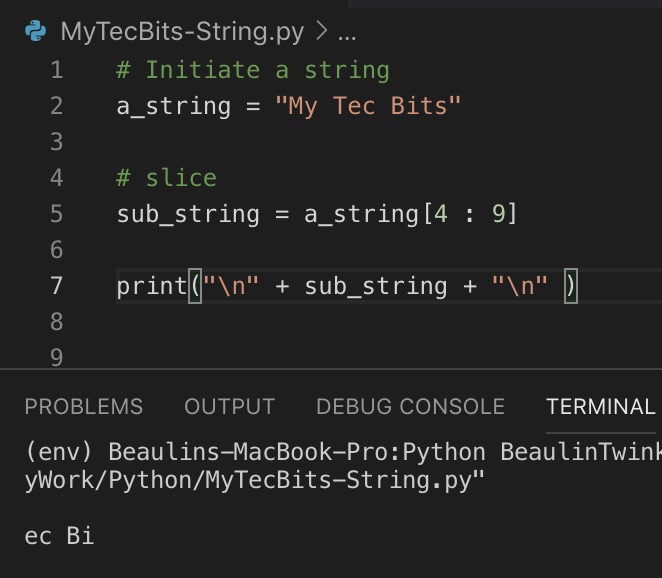
More Tips
Reference
- More about string slicing in Python docs.