I have recently encountered a task that requires me to retrieve a list of all files in a particular folder. To be clear, I only needed to collect files, not any subdirectories within the folder. Fortunately, I was able to implement a straightforward approach to accomplish this.
My solution involved using the pathlib and os.listdir libraries to scan the specified directory and identify any files. By iterating through the items in the directory and checking each one with pathlib.Path(file_path).is_file(), I was able to confirm if the item was a file. If it was, I added it to a list variable for later use.
This method ensures that only files are included in the list and any subdirectories or other non-file items are excluded. With this list of files, I was able to proceed with my task efficiently and effectively.
List all the files in a directory
from os import listdir
from pathlib import PurePath, Path
folderPath = Path("samples")
listOfFiles = [file for file in listdir(folderPath) if Path.is_file(PurePath.joinpath(folderPath, file))]
print(listOfFiles)
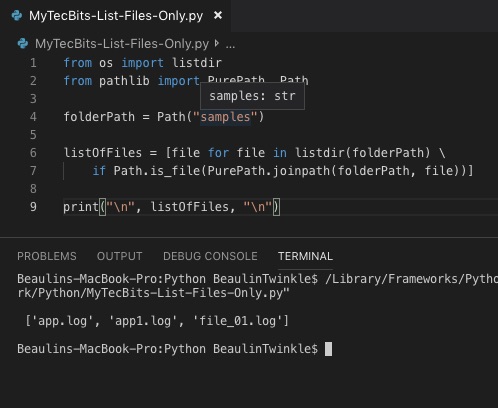
Reference
- About os.listdir at Python Docs.