In Python programming, there are several ways to find the index of an item in a list. Here we will go through some of the most commonly used methods. Let’s go through them one by one.
1. Using the index() method
The easiest and most straightforward way to find the index of an item in a list is to use the built-in index() method. Here is an example:
month_list = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec']
index = month_list.index('Apr')
print(index)
Output
3
The index() method returns the index of the first occurrence of the specified item in the list. This method cannot be used if you want to find every occurrence of a given item.
NOTE: If the item is not found in the list, the index() method will raise a ValueError.
2. Using a for loop
Another way to find the index of an item in a list is to loop through the list and compare each element to the target item. When the item is found, the loop can be terminated, and the index can be returned. Here is an example:
month_list = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec']
target = 'Apr'
for i in range(len(month_list)):
if month_list[i] == target:
print(i)
break
Output
3
This method is useful if you need to find the index of the last occurrence of the item or if you want to perform additional processing while searching for the item.
3. Using list comprehension
List comprehension can be used to generate a list of indices where the item occurs. Let us see an example:
some_list = ['Jan', 'Feb', 'Feb', 'Mar', 'Apr', 'Apr', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec']
target = 'Apr'
indices = [i for i in range(len(some_list)) if some_list[i] == target]
print(indices)
Output
[4, 5, 6]
This method will be very useful if you want to find all occurrences of the given item in the list.
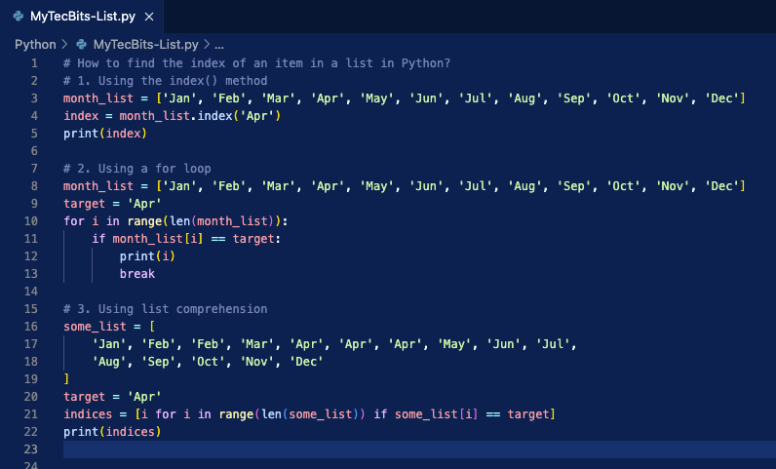
Related Articles
- Get the last element of a list in Python
- How to find the number of elements in a list in Python?
- Check if the list is empty in python
Reference
- More about list and index() method at Python Docs.