Problem
Recently one of my colleague identified a bug in the Python application block. The issue was whenever any change done in a child list is also reflecting in the master list. On reviewing the code, we saw the child list was created by using list_child = list_master method. list = list should not be used to copy or clone. Doing so will not copy the contents of one list to another. Instead the child list will be a reference to the master list. So, any change in the child list will reflect the master list. Let’s see this with an example.
Example
In this example, I have initialized a list named list_master and assigned it to list_child. After removing some items from the list_child, check the list_master. You will see the same elements are removed from the list_master as well. This is because the list_child is just the reference to the list_master not a copy.
# initialize a master list
list_master = [2010, 2011, 2012, 2013, 2014, 2015, 2016, 2017, 2018, 2019, 2020]
# assign master list to a child
list_child = list_master
# pring bith the lists
print("\nPrint master and the child list.")
print(list_child)
print(list_master)
# Remove some elements from the child list
list_child.remove(2010)
list_child.remove(2011)
list_child.remove(2012)
list_child.remove(2013)
# pring bith the lists
print("\nPrint master and the child list after removing some elements from child")
print(list_child)
print(list_master)
Result
Print master and the child list.
[2010, 2011, 2012, 2013, 2014, 2015, 2016, 2017, 2018, 2019, 2020]
[2010, 2011, 2012, 2013, 2014, 2015, 2016, 2017, 2018, 2019, 2020]
Print master and the child list after removing items from child
[2014, 2015, 2016, 2017, 2018, 2019, 2020]
[2014, 2015, 2016, 2017, 2018, 2019, 2020]
Solution
To avoid this problem you have to use the list.copy() method or the list() method or the copy.copy() method. Here are the examples.
(1) Using list.copy()
list_master = [2010, 2011, 2012, 2013]
# Copy the master list to a child
list_child = list_master.copy()
# Remove some elements from the child list
list_child.remove(2010)
list_child.remove(2011)
print(list_child)
print(list_master)
Result
[2012, 2013]
[2010, 2011, 2012, 2013]
(2) Using list()
import copy
list_master = [2010, 2011, 2012, 2013]
# Copy the master list to a child
list_child = copy.copy(list_master)
# Remove some elements from the child list
list_child.remove(2010)
list_child.remove(2011)
print(list_child)
print(list_master)
Result
[2012, 2013]
[2010, 2011, 2012, 2013]
Using list()
list_master = [2010, 2011, 2012, 2013]
# Copy the master list to a child
list_child = list(list_master)
# Remove some elements from the child list
list_child.remove(2010)
list_child.remove(2011)
print(list_child)
print(list_master)
Result
[2012, 2013]
[2010, 2011, 2012, 2013]
Reference
- about list on Python docs.
- More Python tips.
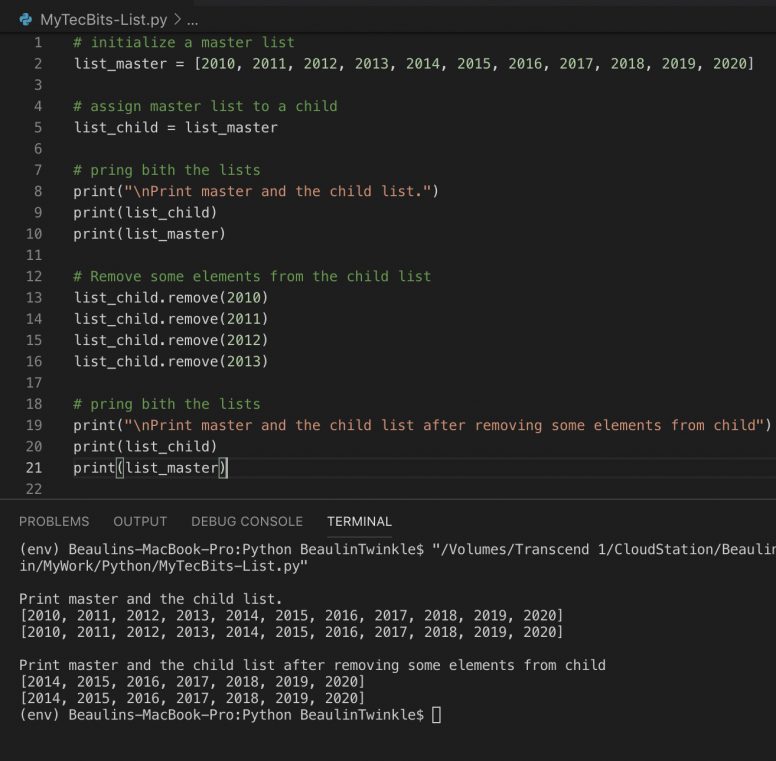