In an earlier article we have seen how to check if the file exists or not. Now we will see how to open the file, read the contents to a list variable with a line per list item. Reading a file into a list is quiet simple in python. You have to use the open() built-in function and the readlines() function. Here is an example.
from pathlib import Path
file = Path("samples/app.log")
if file.is_file():
file_content_list = open(file).readlines()
print("\n", file_content_list)
else:
print("\nFile does not xists.\n")
In the above example, each and every item of the list will have the ending line break (\n). To remove this, use [item.rstrip(“\n”) for item in file_content_list]. Here is the sample with line break symbol removal code.
from pathlib import Path
file = Path("samples/app.log")
if file.is_file():
file_content_list = open(file).readlines()
file_content_list = [item.rstrip("\n") for item in file_content_list]
print("\n", file_content_list)
else:
print("\nFile does not xists.\n")
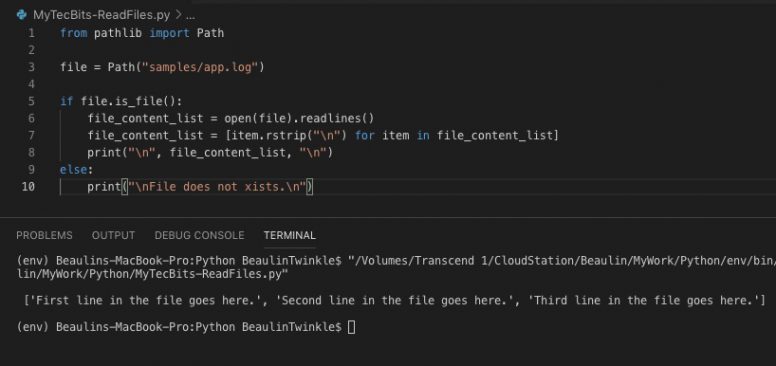
You may also like to read about importing a CSV file to a variable in Python.
Reference
- More about the in-built open() function at Python docs.