You may need your PHP script to sleep or pause for a few seconds or microseconds or nanoseconds to avoid synchronization issues. By default, PHP has several functions like sleep(), usleep(), time_nanosleep(), time_sleep_until(), etc.. to pause the script for some time.
The sleep() function is used for pausing the script for a time duration in seconds. On the other hand, usleep() is used for pausing the script in microseconds. The time_namosleep() function takes in both seconds and nanoseconds to pause the script, so as you can precisely specify the duration in seconds and nanoseconds. Finally the time_sleep_until() function helps you to pause the script until a specific time.
Sleep in seconds
As mentioned before, you can use the sleep() function, if you want to make the PHP script to sleep for a few seconds. Her his an example:
<?php
echo 'Time Before Sleep: ' . date('h:i:s a', time()) . ' <br/>';
Sleep(3);
echo 'Time After Sleep: ' . date('h:i:s a', time()) . ' <br/>';
?>
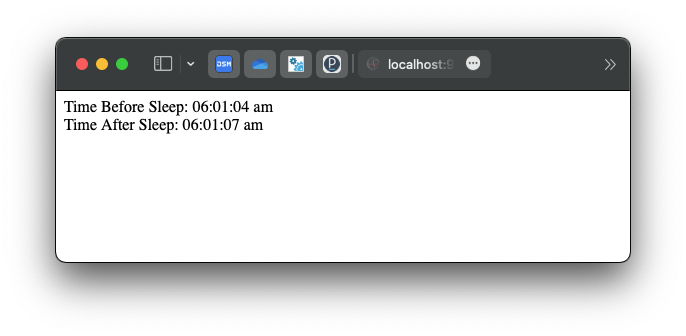
Sleep in microseconds
For making the PHP script to pause in microseconds, then use the function usleep(). Here is an example:
<?php
echo 'Time Before usleep: ' . microtime(true) . ' <br/>';
usleep(20000); // Sleep for 20,000 microseconds.
echo 'Time After usleep: ' . microtime(true) . ' <br/>';
?>
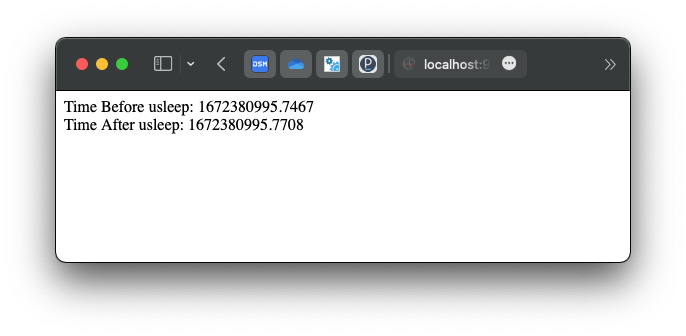
Sleep in seconds & nanoseconds
In case if you want to make the script for a few seconds and nanoseconds then use the function time_nanosleep().
Syntax
time_nanosleep(int $seconds, int $nano_seconds);
Example
<?php
echo 'Time Before Sleep: ' . microtime(true) . ' <br/>';
$seconds = 2;
$nano_seconds = 50;
time_nanosleep($seconds, $nano_seconds);
echo 'Time After Sleep: ' . microtime(true) . ' <br/>';
?>
Sleep till a specific time
To pause a script till a specific timestamp, use the time_sleep_until() function. Here is an example.
<?php
echo 'Time Before Sleep: ' . date('h:i:s a', time()) . ' <br/>';
time_sleep_until(time() + 2);
echo 'Time After Sleep: ' . date('h:i:s a', time()) . ' <br/>';
?>
Related Article
- About pausing a Python script.
Reference
- More about sleep() function at PHP Manual.
- More about usleep() function at PHP Manual.