In the previous articles we have seen how to merge two lists. Now we will see how to merge two dictionaries. There are several methods and workarounds available for merging two dictionaries in Python language. Few of them are based on the version of the Python you are using. Here are three simple and commonly used dictionary merging methods.
Method 1: Using union operator (|)
In Python version 3.9 and higher, you can use the union operator (|) to merge two dictionaries. Here is the syntax and examples to merge dictionaries using union operator (|).
Syntax
c = a | b
Example
>>> a = {1: 'Tiger', 2: 'Leopard'}
>>> b = {3: 'Jaguar', 4: 'Panther'}
>>> c = a | b
>>> print(c)
{1: 'Tiger', 2: 'Leopard', 3: 'Jaguar', 4: 'Panther'}
>>>
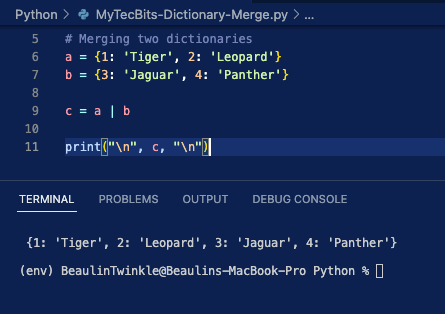
Example for merging more than two dictionaries
>>> a = {1: 'Tiger', 2: 'Leopard'}
>>> b = {2: 'Jaguar', 3: 'Panther'}
>>> c = {3: 'Cat', 4: 'Dog'}
>>> d = a | b | c
>>> print(d)
{1: 'Tiger', 2: 'Jaguar', 3: 'Cat', 4: 'Dog'}
>>>
Method 2: Using kwargs (**)
You can use kwargs (**) to merge two dictionaries in Python version 3.5 and higher. Here is the syntax and examples.
Syntax
c = {**a, **b}
Example
>>> a = {1: 'Tiger', 2: 'Leopard'}
>>> b = {3: 'Jaguar', 4: 'Panther'}
>>> c = {**a, **b}
>>> print(c)
{1: 'Tiger', 2: 'Leopard', 3: 'Jaguar', 4: 'Panther'}
>>>
Example for merging more than two dictionaries
>>> a = {1: 'Tiger', 2: 'Leopard'}
>>> b = {2: 'Jaguar', 3: 'Panther'}
>>> c = {3: 'Cat', 4: 'Dog'}
>>> d = {**a, **b, **c}
>>> print(d)
{1: 'Tiger', 2: 'Jaguar', 3: 'Cat', 4: 'Dog'}
>>>
Method 3: Using update()
If you are using Python version 3.4 or lower, then use the update() to merge two dictionaries. Here is the syntax and examples.
Syntax
a.update(b)
Example to merge one dictionary to another
>>> a = {1: 'Tiger', 2: 'Leopard'}
>>> b = {3: 'Jaguar', 4: 'Panther'}
>>> a.update(b)
>>> print(a)
{1: 'Tiger', 2: 'Leopard', 3: 'Jaguar', 4: 'Panther'}
>>>
Example to merge two dictionaries and create a new dictionary
If you want to create a new dictionary with the values merged from two existing dictionaries, then you have to use the copy() method along with the update() method. Here is the sample code.
>>> a = {1: 'Tiger', 2: 'Leopard'}
>>> b = {3: 'Jaguar', 4: 'Panther'}
>>> c = a.copy()
>>> c.update(b)
>>> print(c)
{1: 'Tiger', 2: 'Leopard', 3: 'Jaguar', 4: 'Panther'}
>>>
Example to merge more than two dictionaries and create a new dictionary
>>> a = {1: 'Tiger', 2: 'Leopard'}
>>> b = {2: 'Jaguar', 3: 'Panther'}
>>> c = {3: 'Cat', 4: 'Dog'}
>>> d = a.copy()
>>> d.update(b)
>>> d.update(c)
>>> print(d)
{1: 'Tiger', 2: 'Jaguar', 3: 'Cat', 4: 'Dog'}
>>>
Reference
- Read more about merging dictionaries using union operator in Python PEP.