Here is one among the simple ways for reading XML files from PHP code. Here I’ve used the SimpleXML XML manipulation function of PHP to read the XML and then using a foreach loop to iterate through the nodes and display the values.
Tools Used
- PHP 7.3.29
- macOS Big Sur
- Visual Studio Code
XML file
This is the xml file team.xml inside the folder team.
<?xml version="1.0" encoding="UTF-8"?>
<team_members>
<member>
<name>Walter White</name>
<designation>Chief Executive Officer</designation>
<image_file>team-1.jpg</image_file>
<linkedin></linkedin>
<twitter></twitter>
<facebook></facebook>
<about>Something</about>
</member>
<member>
<name>Sarah Jhinson</name>
<designation>Product Manager</designation>
<image_file>team-2.jpg</image_file>
<linkedin></linkedin>
<twitter></twitter>
<facebook></facebook>
<about></about>
</member>
<member>
<name>William Anderson</name>
<designation>CTO</designation>
<image_file>team-3.jpg</image_file>
<linkedin></linkedin>
<twitter></twitter>
<facebook></facebook>
<about>Something else</about>
</member>
<member>
<name>Amanda Jepson</name>
<designation>Accountant</designation>
<image_file>team-4.jpg</image_file>
<linkedin></linkedin>
<twitter></twitter>
<facebook></facebook>
<about>Amanda is honest</about>
</member>
</team_members>
PHP code for reading the XML file
To read the team.xml, I’ve used the SimpleXML’s function simplexml_load_file. This function interprets the xml and returns an object. Then the object is iterated using a foreach loop to access all the children for the XML and displays them in a browser.
<?php
$xmlData = simplexml_load_file("team/team.xml")
or die("Unable to load the xml.");
if ($xmlData->count() > 0)
{
foreach($xmlData->children() as $member) {
echo $member->name . ', ';
echo $member->designation . ', ';
echo '<img style="width:50px;" src="/team/'.
$member->image_file . '" alt="' . $member->name . '"> , ';
echo $member->about . '<br/>';
}
}
?>
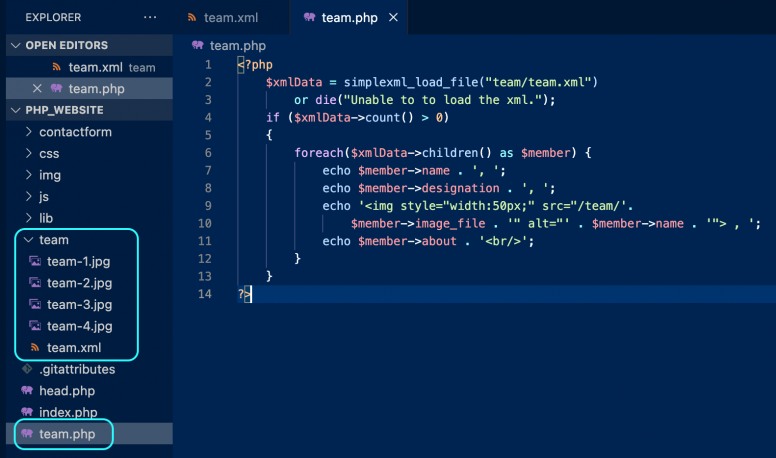
Result
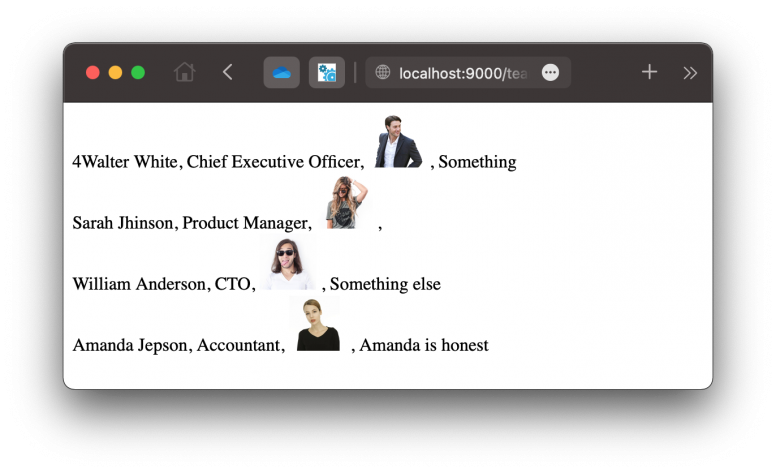
Reference
- More about simplexml_load_file at PHP Manual.
- More about SimpleXML at PHP Manual.
Very useful code. Could you please explain how to use the function to access all of an element’s children? For example, the element Walter White Let’s say that in my XML file, “Walter White” has many variations inside the . Regards.