There are a few different ways to find whether the string contains a substring in a Python program. The easiest method is to use the in operator. Other methods include string.find() and string.index() methods. Let’s see them one by one with examples.
The easiest method: Using “in” operator
As said, the easiest way to find whether the string contains a substring in Python program is by using in operator. The in operator returns a boolean value, either True or False. So, it is best to use it in an if condition. This is the preferred method to find a substring in a string if you do not need to know the position of the substring. Here is an example.
# Initiate a string
a_string = 'My Tec Bits'
sub_string = 'My'
# Check for substring
if (sub_string in a_string) == True:
print('Yes')
else:
print('No')
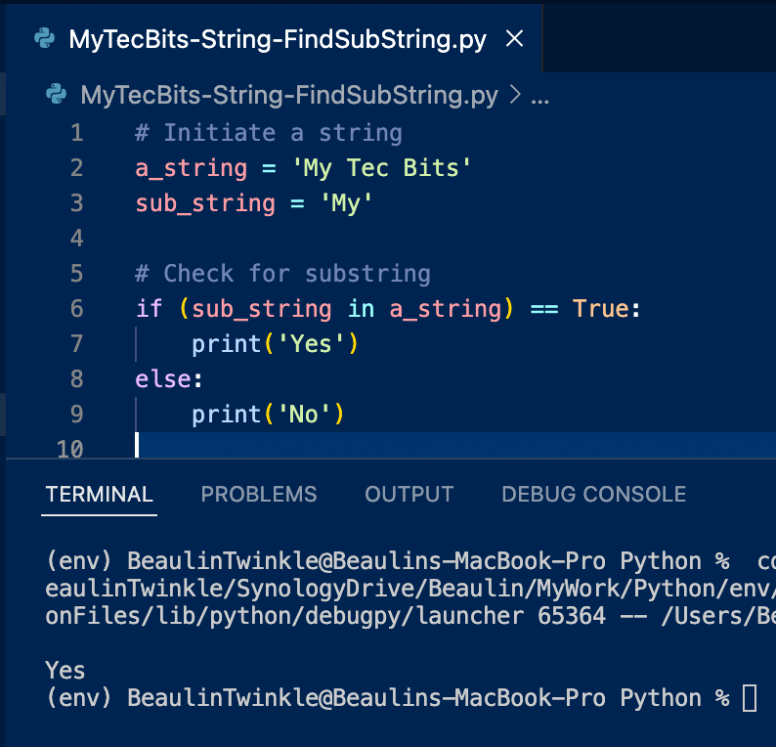
Other Methods
Apart from using the in operator, there are few other methods to find substring in a string. Let us see a couple of these methods using string.find() and string.index() here. These methods are useful if you want to know the position of the substring.
Using string.find() method
The string.find() method searches the given string for a specific sub string and returns the position of where the sub string was found first. If the substring is not found, then the find() method returns -1. It returns 0 if the substring is found at the beginning. So, we can use the find() method in a if condition and check if the value is 0 or more to say whether the substring is available in the mainstring. Here is a sample code:
# Initiate a string
a_string = 'My Tec Bits'
sub_string = 'My'
# Check for substring
if a_string.find(sub_string) >= 0:
print('Yes')
else:
print('No')
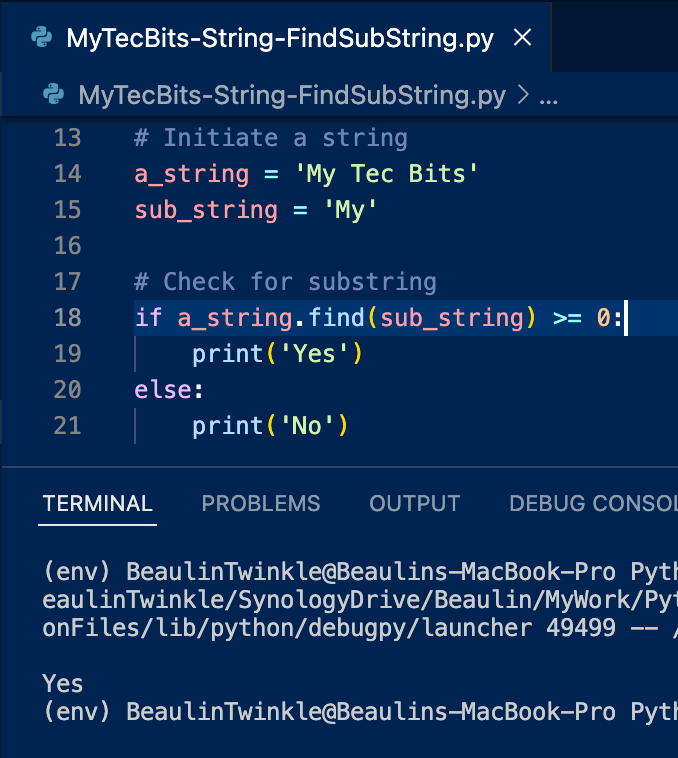
Using string.index() method
Just like str.find() the string.index() method searches the given string for a specific substring and returns the position of where it was found first. However, unlike str.find() the str.index() method throws an exception if the substring is not found in the main string. So, you have to handle the exception if you are using str.index() to look for the substring. Here is an example:
# Initiate a string
a_string = 'My Tec Bits'
sub_string = 'My'
# Check for substring
try:
a_string.index(sub_string)
except ValueError:
print('No')
else:
print('Yes')
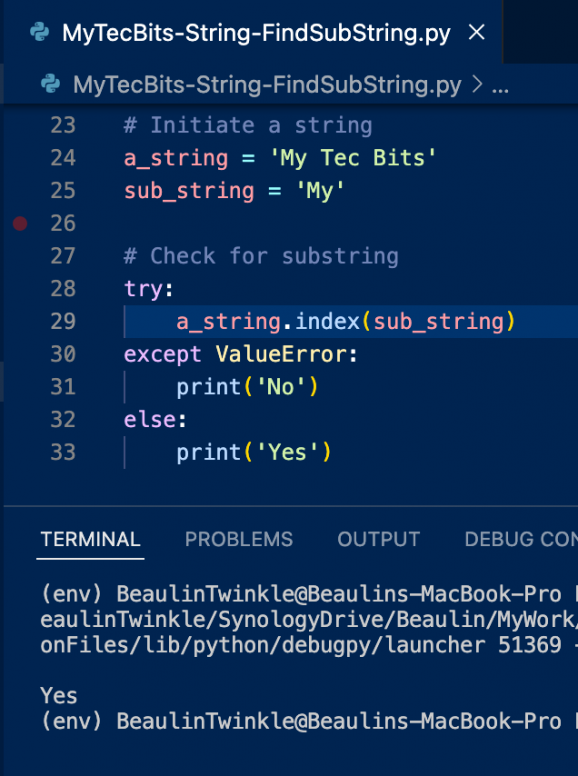
In case if you are interested in getting a specific sub-string from a string in Python, then read my other article Get A Sub-String From A String In Python.
Reference
- More about string.find() at Python Docs.
- More about string.index() as Python Docs.