In one of my previous articles, we have seen how to extract numbers from a string in Python. Now we will see how to check if the given string is a number or not.
Python provides many built-in functions that simplify the task of performing various operations. One of these operations is to check if a given string is a number or not. In this article, we will discuss how to perform this task using various methods in Python.
Method 1: Using the str.isdigit() method
The str.isdigit() method is a built-in function in Python that checks if the given string’s characters are all digits or not. If all the characters are digits, it returns True; otherwise, it returns False. We can use this method to check if a given string is a number or not.
Example
string1 = "0123456"
string2 = "0123456abcde"
string3 = "abcde"
print(string1.isdigit())
print(string2.isdigit())
print(string3.isdigit())
Output
True
False
False
In the above example, we have used the isdigit() method to check if string1, string2 and string3 are numbers or not. string1 contains only digits so it returns True. string2 contains both digits and alphabets so it returns False. string3 contains only alphabets so it also returns False.
Method 2: Using the try-except block
We can also check if a given string is a number or not by using the try-except block. In this method, we try to convert the string to a number using the int() or float() function. If the conversion is successful, then the string is a number; otherwise, it is not.
Example
string1 = "0123456"
string2 = "0123456abcde"
string3 = "abcde"
try:
int(string1)
print(string1 + " is a number.")
except ValueError:
print(string1 + " is not a number.")
try:
int(string2)
print(string2 + " is a number.")
except ValueError:
print(string2 + " is not a number.")
try:
int(string3)
print(string3 + " is a number.")
except ValueError:
print(string3 + " is not a number.")
Output
0123456 is a number.
0123456abcde is not a number.
abcde is not a number.
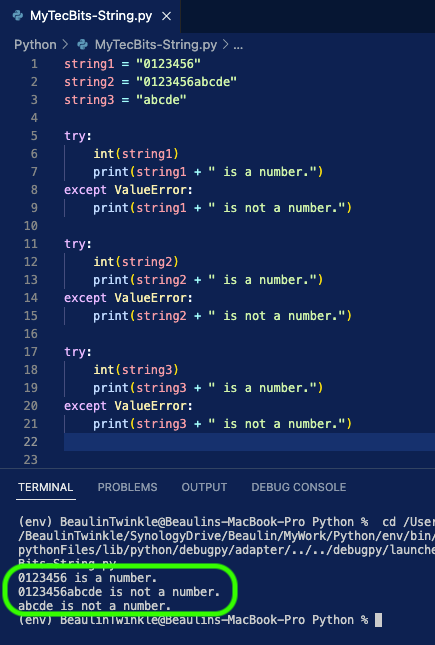
In the above example, we have used the try-except block to check if the given strings are numbers or not. string1 can be converted to an integer so it is a number. string2 cannot be converted to an integer because it contains alphabets so it is not a number and raises a ValueError. string3 also cannot be converted to an integer because it contains only alphabets.
Reference
- More about str.isdigit() at Python Docs.