In Python, functions are essential building blocks. They allow you to encapsulate reusable pieces of code. One powerful feature of functions is the ability to return values, which can be especially useful when you want to retrieve multiple pieces of data from a single function call. This article delves into the few common methods available for returning multiple values from a function, illustrating how Python provides flexibility in this aspect, similar to other programming languages.
1. Returning Objects
One approach to return multiple values from a function is to encapsulate the values into an object, such as a custom class instance. This method is particularly useful when the returned values are related and have a logical connection. By packaging them into an object, you maintain encapsulation and can access the values through the object’s attributes or methods.
Here’s an example demonstrating how to use objects to encapsulate and return multiple values from a function:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def get_person_details():
name = "Alice Deo"
age = 30
person = Person(name, age)
return person
person_info = get_person_details()
print(f"Name: {person_info.name}")
print(f"Age: {person_info.age}")
In this example, we define a Person class with attributes name
and age
. The get_person_details function creates an instance of the Person class and sets its attributes with the desired values. Then, it returns the person object, encapsulating both the name and age. When we call get_person_details() and receive the returned object, we can access its attributes (name and age) using dot notation, just like any other object attributes.
2. Returning Tuples
Tuples are lightweight, ordered collections in Python that can hold multiple items of different types. A common practice is to return a tuple from a function, where each element of the tuple corresponds to a different value. This method allows you to return heterogeneous data in a structured manner. Tuples are immutable, ensuring that the returned values remain consistent.
Here’s an example on how to use tuples to retrieve multiple values from a function:
def get_person_details():
name = "Alice Deo"
age = 30
return name, age
person_info = get_person_details()
print(f"Name: {person_info[0]}")
print(f"Age: {person_info[1]}")
In this example, the function get_person_details returns a tuple containing the person’s name and age. The values are accessed using indexing (person_info[0] and person_info[1] which corresponds to the order in which they are returned by the function.
3. Returning Lists
Returning a list from a function is another approach to providing multiple values as output. While similar to returning a tuple, lists offer the advantage of mutability. You can modify the list’s contents after it’s returned, which can be beneficial in certain scenarios. However, remember that mutability introduces the potential for unintended changes, so caution is necessary.
Here’s an example on how to use the lists to retrieve multiple values from a function:
def get_person_details():
name = "Alice Deo"
age = 30
return [name, age]
person_info = get_person_details()
print(f"Name: {person_info[0]}")
print(f"Age: {person_info[1]}")
In this example, the get_person_details function returns a list containing the person’s name and age. The returned list is assigned to the variable person_info, and we access each value using indexing (person_info[0] and person_info[1]).
4. Returning Dictionaries
Dictionaries are key-value pairs that enable efficient data retrieval based on unique keys. Returning a dictionary from a function allows you to associate each value with a descriptive key. This method is particularly useful when you want to provide context for the returned values or when the order of the values is not significant.
Here is an example:
def get_person_details():
name = "Alice Deo"
age = 28
return {"name": name, "age": age}
person_info = get_person_details()
print(f"Name: {person_info['name']}")
print(f"Age: {person_info['age']}")
In this example, the get_person_details function returns a dictionary with keys “name” and “age” representing the person’s information. The returned dictionary is assigned to the variable person_info, and we access each value using dictionary indexing (person_info[‘name’] and person_info[‘age’]).
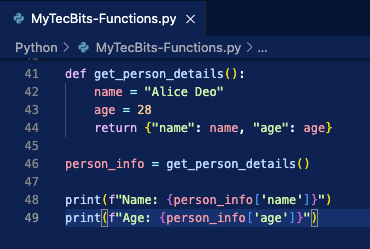
Conclusion
Ultimately, the choice of which method to use for returning multiple values from a function depends on the specific requirements of your code and the relationships between the values. Python’s flexibility enables you to adopt the approach that best suits your needs. By understanding these different techniques, you can enhance the readability and efficiency of your Python code while efficiently extracting multiple values from your functions.
Reference
- Read more about functions at Python Docs.