In our previous article, we explored how to encode a given string to ASCII in C#, an essential process for converting text into binary data. Now, let’s dive into the inverse operation – decoding ASCII back to text in C#.
Decoding ASCII to text is crucial for various scenarios. For instance, when reading ASCII files, processing legacy data, or handling data transmitted in ASCII format, the decoding process ensures the data can be easily understood and manipulated.
To decode ASCII to text in C#, you can use the Encoding.ASCII class along with its GetString method. The Encoding.ASCII class provides functionality to convert a byte array containing ASCII-encoded data back into a readable text string. Here’s an example:
using System;
using System.Text;
class Program
{
static void Main()
{
byte[] asciiBytes = { 72, 101, 108, 108, 111, 44, 32, 77, 121, 84, 101, 99, 66, 105, 116, 115, 33 };
ASCIIEncoding asciiEncoding = new ASCIIEncoding();
// Step 1: Decode ASCII to Text
string decodedText = asciiEncoding.GetString(asciiBytes);
Console.WriteLine("Decoded Text: " + decodedText);
}
}
Result:
Decoded Text: Hello, MyTecBits!
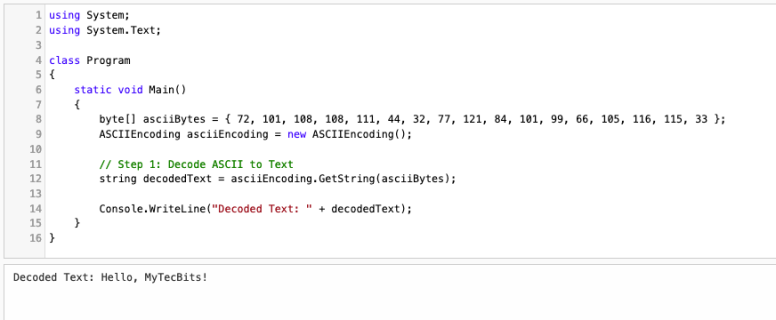
In the provided example, the ASCII encoded value “72, 101, 108, 108, 111, 44, 32, 77, 121, 84, 101, 99, 66, 105, 116, 115, 33” is initially stored into a byte array called asciiBytes. Each individual ASCII value is split, converted, and sequentially placed in the byte array. This array now holds the ASCII-encoded data.
Subsequently, the ASCIIEncoding.GetString() method comes into play, performing the essential decoding task. By passing the asciiBytes array as an argument, the method intelligently converts the ASCII-encoded bytes back into human-readable text. As a result, the decoded text “Hello, MyTecBits!” is obtained, mirroring the original string before the encoding process.
In summary, the process effectively reverses the encoding operation, allowing for seamless data transformation between ASCII-encoded binary and easily interpretable text representations.
You can check our online ASCII Decoder Tool here. We have used the above C# code to develop this tool. It will decode ASCII encoded bytes to readable text format.
Reference
- Read more about Encoding.ASCII property at Microsoft Docs.