In my previous article, we have see how to calculate statistical median in C#.NET. Now we will see how to calculate arithmetic mean in C#. Arithmetic mean is nothing but the average of the collection of numbers. It is calculated by adding all the numbers in the collection and divided by the count of numbers in the collection. So, calculating mean in C# is quite straightforward. There are several ways to find mean in C#. Here are a few of the methods.
Write Your Own Code
To start with we will see a sample of implementing the algorithm of arithmetic mean by coding yourself. In this, I wrote a function GetMean which takes in a single dimensional array of double data type as . Using foreach loop, iterate through the array to get the sum of all the elements of the array. Then divide it with the number of elements in the array.
public static double GetMean(double[] arrSource)
{
// Check if the array has values
if (arrSource == null || arrSource.Length == 0)
throw new ArgumentException("Array is empty.");
// Calculate the mean
double sumOfArray = arrSource.Sum();
return sumOfArray / arrSource.Length;
}
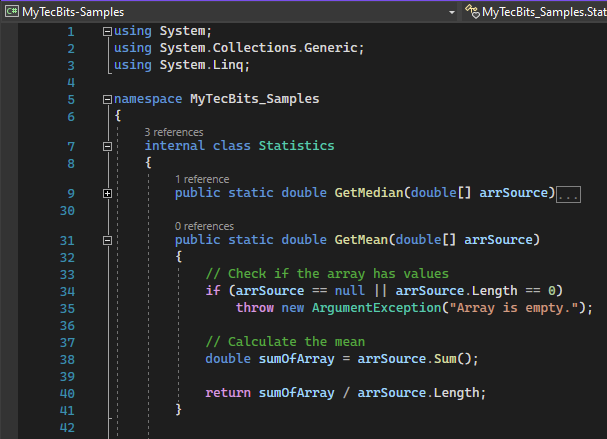
Using Linq
Next method is by using Linq to find the average of the numbers in an array. If you are using Linq in your application, then this would be the right choice for you. You just need to use the Linq’s Average method. Here is an example:
using System.Linq;
...
...
double[] arrSource = {1, 23, 45, 56. 83.5, 96, 5.2, 3};
double mean = 0;
mean = arrSource.Average();
Using third-party library
Finally, finding mean using the third-party library MathNet.Numerics. In the MathNet.Numerics.Statistics namespace there is method Mean() to find the arithmetic mean. Here is how to use it.
using MathNet.Numerics.Statistics;
...
...
double[] arrSource = {1, 23, 45, 56. 83.5, 96, 5.2, 3};
double mean = 0;
mean = arrSource.Mean();
Related articles
- Read about calculating statistical Median in SQL Server.
- Read about calculating arithmetic Mean In SQL Server.
- Read about calculating Mode In SQL Server.
Reference
- More about arithmetic mean at Wikipedia.