In my previous articles, I wrote about calculating statistical median and arithmetic mean in C#.NET. Now we will see how to calculate mode in C#.
In mathematical statistics, the value which appears most often in the given data set is called mode of the data set. Based upon the number of modes, a data set can have one mode (unimodal) or multiple modes (bimodal, trimodal or multimodal).
In C#.NET, there is no inbuilt method to find mode. So, we have to write our own code to find mode or use a third party library. One of the easiest ways I Am using to find the mode of the given set of numbers is by using LINQ. Here is the sample code to calculate mode of a set of numbers in an array in C#. This method will get you single mode or multiple modes.
NOTE: In this method, If there is no mode in the given set, then the function will return all the numbers in the set. If you want to avoid this, then add an if condition to compare the number of elements in the source array and the result. If the count is equal then return an empty array.
using System;
using System.Collections.Generic;
using System.Linq;
namespace MyTecBits_Samples
{
internal class Statistics
{
public static IEnumerable<double> GetMode(double[] arrSource)
{
// Check if the array has values
if (arrSource == null || arrSource.Length == 0)
throw new ArgumentException("Array is empty.");
// Convert the array to a Lookup
var dictSource = arrSource.ToLookup(x => x);
// Find the number of modes
var numberOfModes = dictSource.Max(x => x.Count());
// Get only the modes
var modes = dictSource.Where(x => x.Count() == numberOfModes).Select(x => x.Key);
return modes;
}
}
}
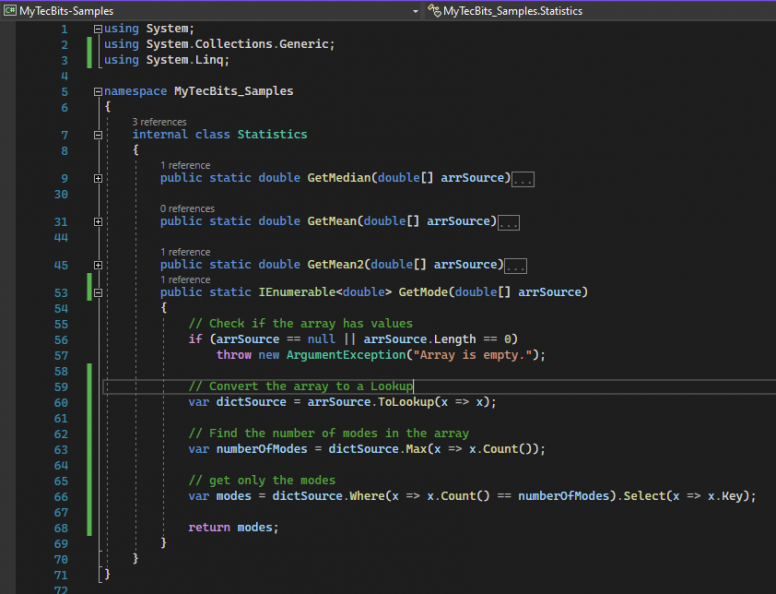
If interested, you can checkout our online Mean, Median & Mode calculator developed in .NET.
Related Articles
- Read about calculating statistical Median in SQL Server.
- Read about calculating arithmetic Mean In SQL Server.
- Read about calculating Mode In SQL Server.
Reference
- Read more about statistical mode @ Wikipedia.