While using any website’s URL in programming, it is always safe to use URL Encoding. URL Encoding is also called as Percent-Encoding. This is because the Unsafe ASCII characters in the URL is encoded to % followed by a hexadecimal code. The browsers can recognize the URLs in standard ASCII format. Any specisl charactor needs to be percent-encoded before using in programming.
URL Encoding
Java provides URLEncoder HTML utility class to percent-encode and decode url query string. The method encode in the URLEncoder class is used for percent encoding. This encode method takes in the URL and returns the url encoded string. Let us see an example of how to use the URLEncoder.encode class.
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
public class MTBSampleEncode {
public static void main(String args[]) {
try {
String urlQueryString = "https://www.mytecbits.com/tools/encoders/url-encoder";
String encodedQueryString = URLEncoder.encode(urlQueryString, "UTF-8");
System.out.println("Encoded URL Query String: " + encodedQueryString);
}
catch (UnsupportedEncodingException e) {
System.err.println(e);
}
}
}
The result of above encoding will be https%3a%2f%2fwww.mytecbits.com%2ftools%2fencoders%2furl-encoder
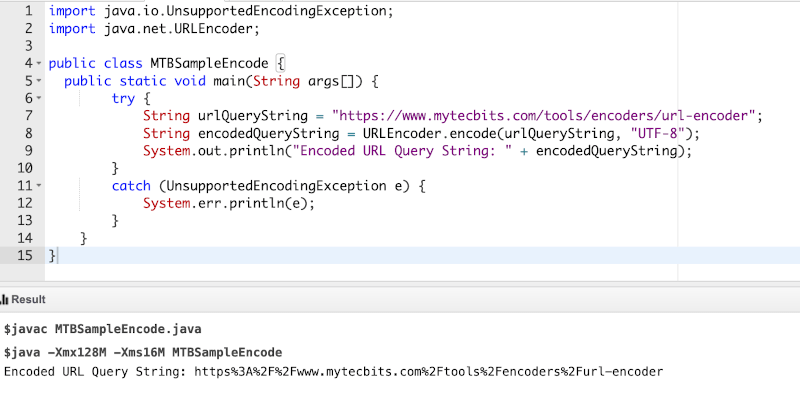
URL Decoding
To decode the already encoded url, Java provides the URLDecoder class. The decode method in the URLDecoder class can be used to get back the regular url. Let us see a sample code.
import java.io.UnsupportedEncodingException;
import java.net.URLDecoder;
public class MTBSampleURLDecode {
public static void main(String args[]) {
try {
String urlQueryString = "https%3a%2f%2fwww.mytecbits.com%2ftools%2fencoders%2furl-encoder";
String decodedQueryString = URLDecoder.decode(urlQueryString, "UTF-8");
System.out.println("Decoded URL Query String: " + decodedQueryString);
}
catch (UnsupportedEncodingException e) {
System.err.println(e);
}
}
}
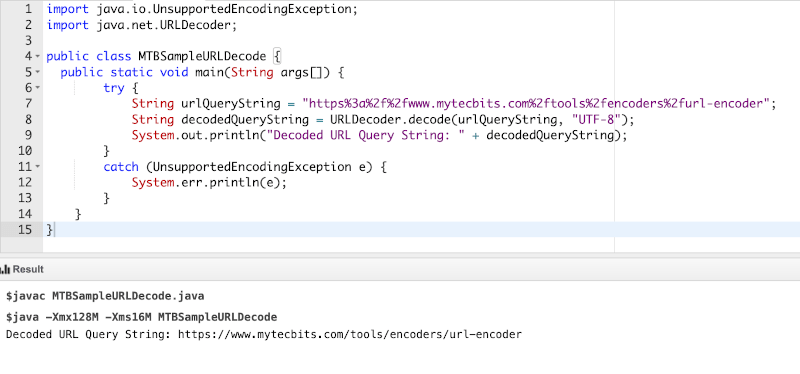
Reference
- Online URL Encoder Tool.
- Online URL Decoder Tool.
- About URLEncoder at Oracle Docs.
- About URLDecoder at Oracle Docs.