In my earlier articles, we have seen how to calculate mathematical statistical median in Sql Server, C# .Net and Python. Now we will see how to calculate the median in PHP.
In PHP, there is no inbuilt method to find the median for an array of numbers. However, you can write your own code to apply the median algorithm to calculate the median. To start with, you have to sort the array. Then, find the midpoint of the array. If the array has an odd number of elements, then the middle element is the median. If the array has an even number of elements, then you have to find the two middle elements and calculate the average of the middle elements to get the mean. Here is the sample code:
Sample code to calculate Median in PHP
<?php
function getMedian($arrInput){
if(is_array($arrInput)){
// Sort the array.
rsort($arrInput);
// Find the middle of count
$size = count($arrInput);
$middle = count($arrInput) / 2;
// Calculate median
if ($size % 2 != 0){
$median = $arrInput[$middle];
}
else{
$median1 = $arrInput[$middle];
$median2 = $arrInput[$middle-1];
$median = ($median1 + $median2) / 2;
}
return $median;
}
}
$array = array(220,100,190,180,250,190,240,180,140,180,190);
echo 'Median: '.getMedian($array).' <br/>';
$array = array(1, 2, 3, 4, 5, 6, 7, 8);
echo 'Median: '.getMedian($array).' <br/>';
$array = array(1, 2, 3, 4, 5, 6, 7, 8, 9);
echo 'Median: '.getMedian($array).' <br/>';
?>
Output
Median: 190
Median: 4.5
Median: 5
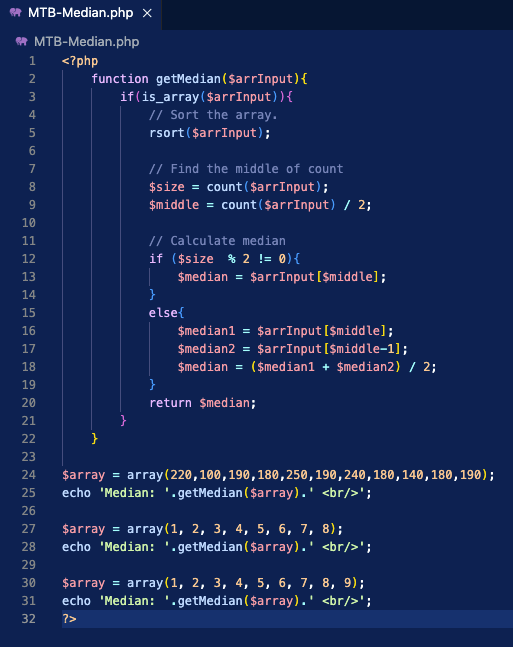
Related Articles
Reference
- Read more about statistical median at WikiPedia.