In my previous articles, I wrote about calculating statistical mode in SQL Server, C#.Net and Python. Now we will see how to calculate mode in PHP.
In mathematical statistics, the value which appears most often in the given data set is called mode of the data set. Based upon the number of modes, a data set can have one mode (unimodal) or multiple modes (bimodal, trimodal or multimodal).
In PHP, there is no inbuilt method to find mode. So, we have to write our own code to find mode or use a third party library. Here is the sample code to calculate the mode of a set of numbers in an array in PHP. This method will get you single mode or multiple modes. If there is no mode then this will return an empty array.
Sample code for calculating Mode in PHP
<?php
function getMode($arrInput){
if(is_array($arrInput)){
// Find the frequency of occurrence of unique numbers in the array
$arrFrequency = [];
foreach( $arrInput as $v ) {
if (!isset($arrFrequency[$v])) {
$arrFrequency[$v] = 0;
}
$arrFrequency[$v]++;
}
// If there is no mode, then return empty array
if( count($arrInput) == count($arrFrequency) ){
return [];
}
// Get only the modes
$arrMode = array_keys($arrFrequency, max($arrFrequency));
return $arrMode;
}
}
$array = array(220,100,190,180,250,190,240,180,140,180,190);
echo 'Mode: ';
print_r (getMode($array));
$array = array(1, 2, 3, 4, 5, 6, 7, 8);
echo '<br/>Mode: ';
print_r (getMode($array));
$array = array(1, 2, 3, 1, 2, 3, 1, 3, 3);
echo '<br/>Mode: ';
print_r (getMode($array));
?>
Output
Mode: Array ( [0] => 190 [1] => 180 )
Mode: Array ( )
Mode: Array ( [0] => 3 )
From the output, you can see the first array has two modes 190 and 180, The second array does not have any modes, So the function returned an empty array. The third array has a single mode 3.
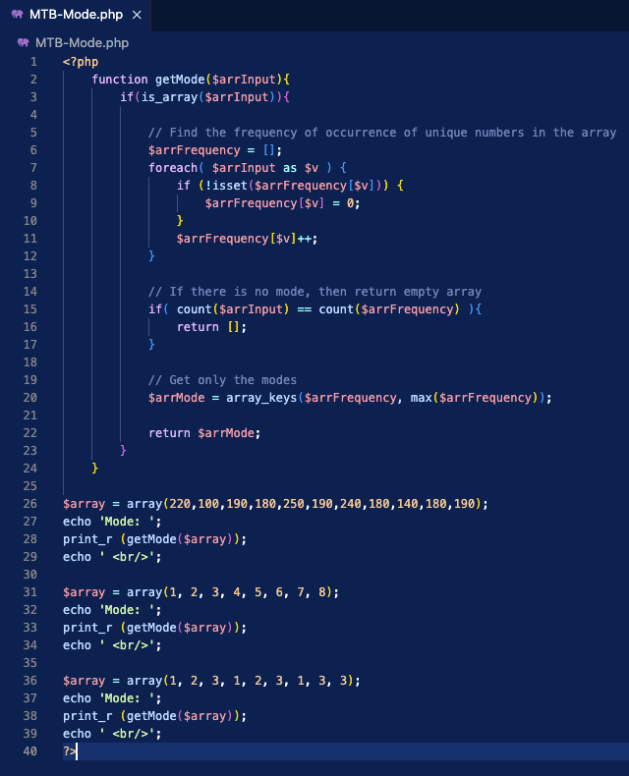
Related Articles
Reference
- Read more about statistical mode @ Wikipedia.