There are multiple ways to delete a file or folder in Python programming. You can use the os module’s remove() method for basic file and directory operations, or you can use the shutil module, which provides more advanced functionality and better cross-platform support. If you have to delete only the empty folders, then you can use the os module’s rmdir() method. Here’s how you can do it using all the three methods:
1. Using the ‘os.remove()’ (for deleting files)
import os
file_path = "mtb/sample_folder/sample_sub_folder/file.txt"
# Check if the file exists before trying to delete it
if os.path.exists(file_path):
os.remove(file_path)
print(f"File {file_path} deleted successfully")
else:
print(f"File {file_path} does not exist")
In this example, we use os.remove(file_path) to delete the specified file. We first check if the file exists using os.path.exists() to avoid errors.
2. Using the ‘shutil’ module (for deleting files and directories)
import os, shutil
folder_path = "mtb/sample_folder"
# Check if the folder exists before trying to delete it
if os.path.exists(folder_path):
shutil.rmtree(folder_path)
print(f"Folder {folder_path} and its contents deleted successfully")
else:
print(f"Folder {folder_path} does not exist")
In this example, we use shutil.rmtree(folder_path) to delete the specified folder and its contents. Again, we check if the folder exists using os.path.exists() to prevent errors.
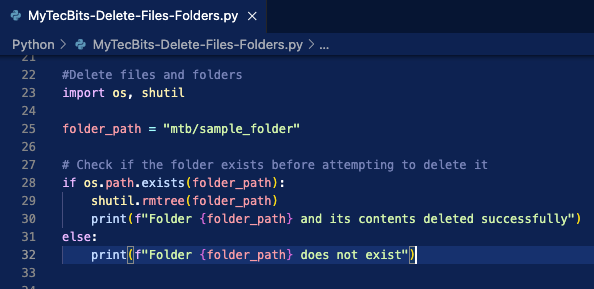
3. Using the ‘os.rmdir()’ (for deleting an empty directory)
The os.rmdir() function in Python is used specifically for deleting empty directories (folders). It will raise an error if you attempt to delete a directory that still contains files or subdirectories.
import os
folder_path = "mtb/sample_folder/sample_sub_folder"
# Check if the folder exists before trying to delete it
if os.path.exists(folder_path):
try:
os.rmdir(folder_path)
print(f"Empty folder {folder_path} deleted successfully")
except OSError as e:
print(f"Error: {e}")
else:
print(f"Folder {folder_path} does not exist")
Please note that os.rmdir() is suitable for deleting empty directories only. If the directory contains any files or subdirectories, it will raise an OSError.
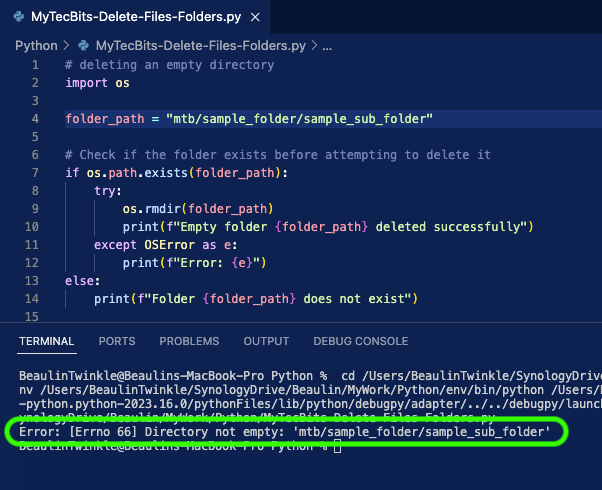
Reference
- Read more about os.remove() at Python Docs.
- Read more about shutil.rmtree() at Python Docs.