To create a directory in Python, including any missing parent directories, you can use the os module or the Path class from the pathlib module. Here’s how you can do it using both methods:
1. Using the ‘pathlib’ module
This method work is Python 3.4 and higher versions. The pathlib module was introduced in Python 3.4, so it will not work in Python versions earlier than 3.4. If you need to work with Python 3 and lower versions (Python 2), you should consider using next methods, using the os
module.
from pathlib import Path
# Specify the directory path you want to create
directory_path = "mtb/path/to/your/directory"
# Create the directory along with any missing parent directories
Path(directory_path).mkdir(parents=True, exist_ok=True)
In this code, the Path class from the pathlib module is used to create a directory. The parents=True argument ensures that any missing parent directories are also created, and exist_ok=True prevents errors if the directory already exists.
2. Using the ‘os’ module
This method works in all the versions of Python even lower than 3.4.
import os
# Specify the directory path you want to create
directory_path = "mtb/path/to/your/directory"
# Create the directory along with any missing parent directories
os.makedirs(directory_path, exist_ok=True)
In this code, os.makedirs() is used to create the specified directory and any missing parent directories. The exist_ok=True argument ensures that the function won’t raise an error if the directory already exists.
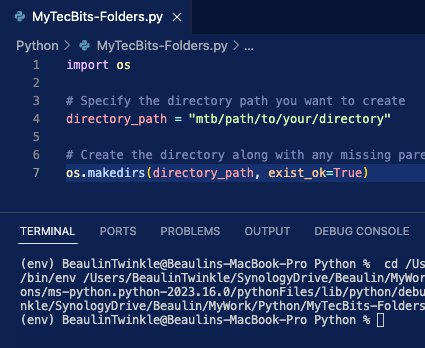
Choose the method that you find more convenient and suitable for your specific use case. Both methods are effective for creating directories and handling missing parent directories.
Reference
- More about os.makedirs at Python Docs.