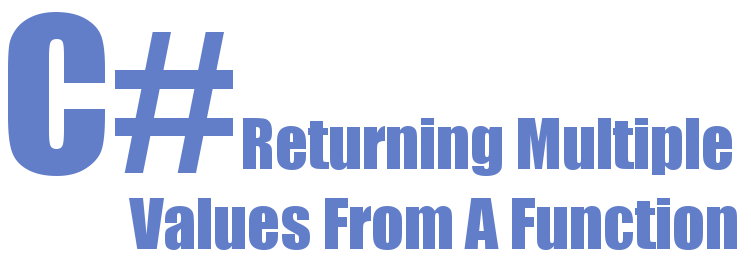
There are several ways to return multiple values of different date types from a c# function. For example, you can use the out parameter, Tuple, Ref, etc.. Here we will see few of them with code samples.
Using Out Variable / Parameter (New Syntax: C# 7.0 and higher)
In C# version 7.0, the out parameter modifier is revamped and made simple to use them. With the new syntax, you can define the out parameter or variable in the method definition and the calling method. Here is an example.
// Method
public void GetReturnValue(out int id, out string name)
{
id = 1034;
name = "John";
}
// Calling the function with out parameter
GetReturnValue(out int id, out string name);
....
....
Using Out Variable / Parameter (Old Syntax)
Prior to C# version 7.0, out parameter must be declared before passing as an argument. Here is an example.
// Method
public void GetReturnValue(out int id, out string name)
{
id = 1034;
name = "John";
}
// Calling the function with out parameter
int id;
string name;
GetReturnValue(out id, out name);
....
....
Using Tuple (New Syntax C# 7.0 and higher)
Another wonderful option is to return multiple values from a method is to use Tuple. In C# version 7, Microsoft introduced, Tuple Deconstruction to use separate variables for tuple. To use tulip deconstruction in your project you have to add reference to the NuGet package System.ValueTuple.
To refer System.ValueTuple from visual studio 2017 project, go to Manage NuGet Packages screen, search and install it.
Then you can use tuple deconstruction as given in the example below.
// Method
public (int id, string name) GetReturnValue()
{
return (103, "David");
}
// Calling the function with tulip
var (id, name) = GetReturnValue();
....
....
Using Tuple (Old Syntax)
If you are using older version of C#, then you have to settle down for the older syntax of Tuple. Here is an example.
// Method
public Tuple<int, string> GetReturnValue()
{
return Tuple.Create(1, "One");
}
// Calling the function with tulip
Tuple<int, string> result = GetReturnValue();
....
....
Using Argument By Reference
Another classic method to return multiple values from a method is by passing argument by reference (using ref keyword). Here is an example.
// Method
public void GetReturnValue(ref int id, ref string name)
{
id = 123;
name = "John";
}
// Calling the function with ref
int id = 0;
string name = "";
GetReturnValue(ref id, ref name);
....
....
Using Structure
One more method to return multiple values from a method is to use the old-fashioned C# structures (struct). Here is an example.
struct Result
{
public int id;
public string name;
}
private Result GetReturnValue()
{
var result = new Result
{
id = 987,
name = "Peter"
};
return result;
}
// Calling the function with struct
Result getResult = GetReturnValue();
MessageBox.Show("ID: " + getResult.id.ToString() + "; Name: " + getResult.name + ";");
....
....
Using Class
Finally, similar to structure, you can use class to return multiple complex values from a C# function or method. Here is an example for returning values using class.
// Define Class
namespace MyTecBits_Samples
{
public class Result
{
public int id;
public string name;
}
}
// Define Method
public Result GetReturnValue()
{
var result = new Result
{
id = 987,
name = "Peter"
};
return result;
}
// Calling the method
Result getResult = GetReturnValue();
MessageBox.Show("ID: " + getResult.id.ToString() + "; Name: " + getResult.name + ";");
....
....